Vertex Webhooks Example
A webhook is a method to provide other applications with real-time information. Unlike other APIs there is no need to poll data to keep it up-to-date.
A webhook delivers data to other applications as it happens, meaning you get data immediately. This makes webhooks much more efficient for both provider and consumer.
For any event in the system you can register a URL which will receive a notification every time an event occurs in your account.
In the tutorial below we will create and send a test webhook.
If you need to use an integration with some of the Payment Gateways mentioned above, just review the appropriate guide.
Configure your server
The server receiving webhooks from the service should respond with a 200 HTTP status code. Any other value will indicate to the service that you did not receive the webhook.
Vertex webhooks are sent via HTTP PUT requests.
When a webhook is not successfully received for any reason, the service will continue trying to send the webhook once an hour for up to 3 days.
The headers and body that are sent back to the service are saved in the database and can be viewed in the Settings / Webhooks / Webhook logs panel:
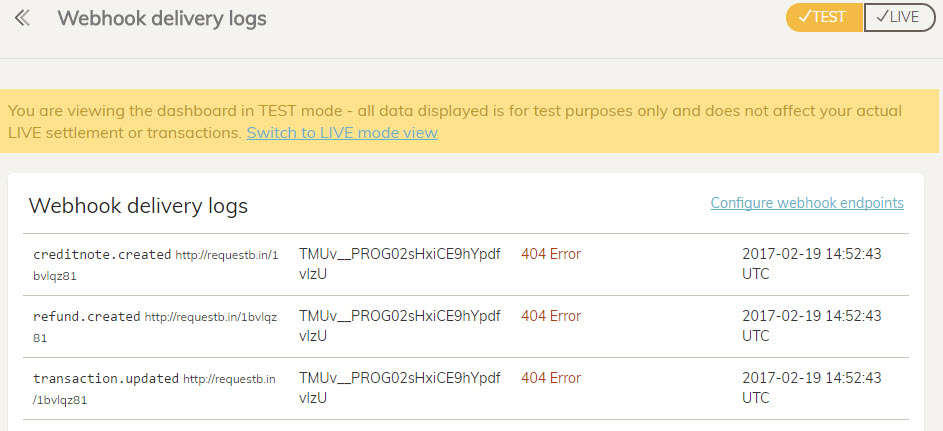
Create a webhook
In this section we will create a sample webhook.
Webhooks are configured in the Settings > Account > Webhooks > Configure webhooks section of the Merchant Portal:
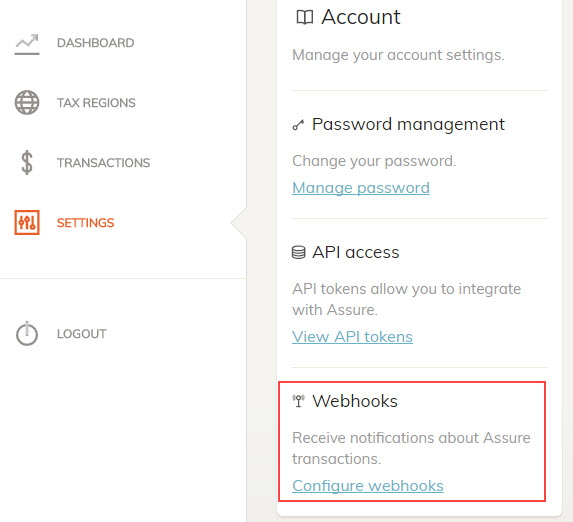
On the Webhooks page:
Click Configure new endpoint
:
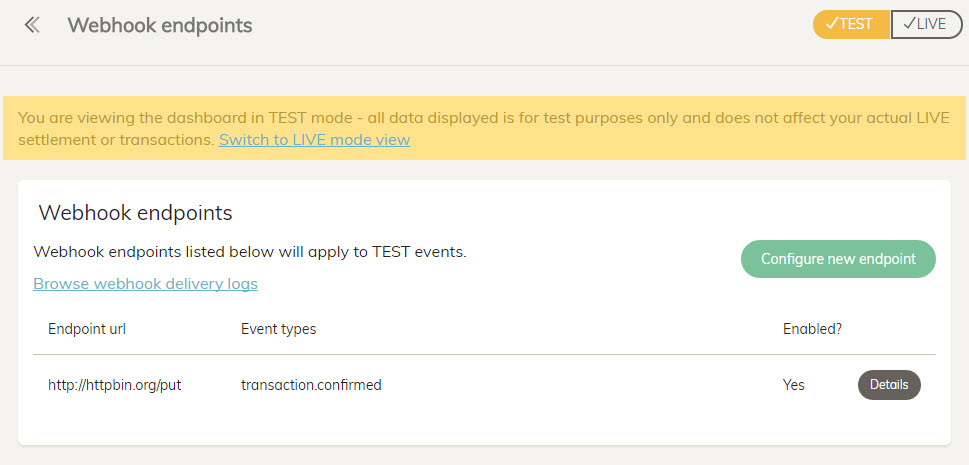
Configure your webhook:
- Set Mode to
TEST
- we don't want to go live yet. - Set the URL to http://httpbin.org/put. It should point to your server but for now we will use the Httpbin public echo server.
- Select the
transaction.confirmed
event type.
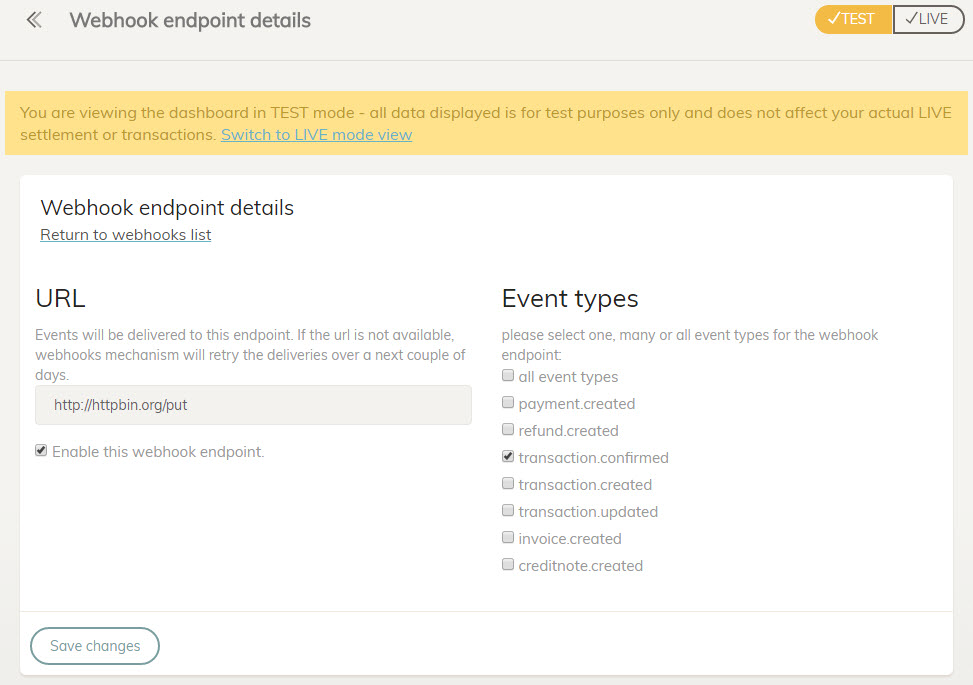
Click Save
. Your new webhook should appear on the list:
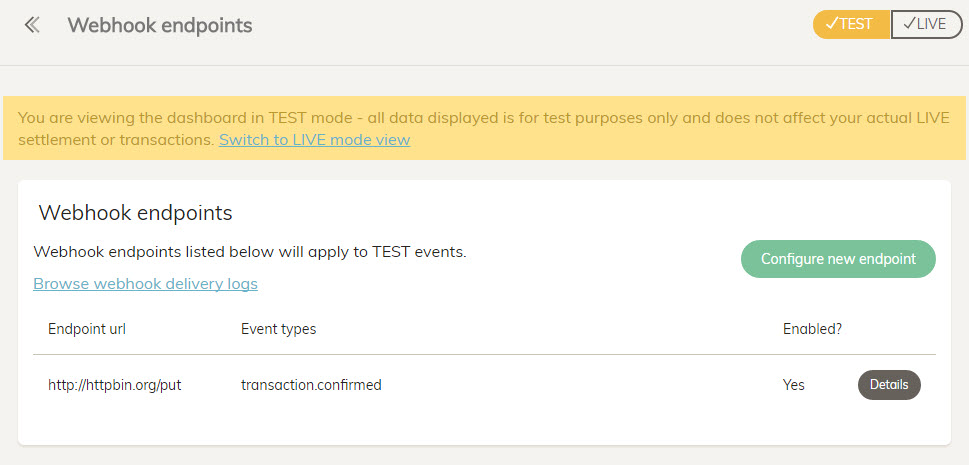
Security
The Vertex webhooks engine has implemented two security mechanisms.
1. Signing events with HMAC-SHA1 hash
All webhook events sent to your server are signed with a HMAC-SHA1 hash generated from the content of the message and a SHA1 hash of your private TEST or LIVE token. This hash may be used to verify the data integrity and the authentication of a message.
The generated hash is sent in a HTTP header in a request to your server.
The process of generating a hash on the service's server goes as follows:
-
Take a corresponding private token of a merchant's account. Use a private LIVE token if the event is sent in LIVE mode, otherwise use a private TEST token.
-
Generate a SHA1 hash from the private token obtained via the above method.
-
Generate a HMAC-SHA1 hash of a webhook message using a SHA1 hash created above as a shared secret key.
-
Add a HTTP header to a HTTP request sent to a merchant's server:
X-Taxamo-Signature: GENERATED-HMAC-SHA1-HASH
You can use the code snippet below to verify the integrity and authenticity of a message received by your server:
<?php
$body = file_get_contents('php://input');
$application_mode = json_decode($body)->{'app_mode'};
switch ($application_mode) {
case "TEST":
$private_token = 'PASTE_YOUR_PRIVATE_TEST_TOKEN_HERE';
break;
case "LIVE":
$private_token = 'PASTE_YOUR_PRIVATE_LIVE_TOKEN_HERE';
break;
}
$actual_signature = $_SERVER['HTTP_X_TAXAMO_SIGNATURE'];
$expected_signature = hash_hmac('sha1', $body, sha1($private_token));
if (strcmp($actual_signature, $expected_signature) == 0) {
echo "Signature is valid!";
} else {
echo "Signature is invalid!";
}
?>
2. Retrieve webhook events from the the service's server
Every time your server receives a webhook event you may want to check whether such a request was sent from the Taxamo server. For such a use case you may use a /api/v2/webhook_events/:event_id
API endpoint to fetch an event with a given id from the Taxamo server.
In order to achieve this you should implement the following scenario:
-
Set up your server to receive webhook notifications from the service (The tutorial below explains how to do this).
-
When your server receives a HTTP request then use an
event_id
from the incoming notification to fetch this event from the Taxamo server. -
If a response code from the service is
200
then you can assume that this event is sent from the service and is not forged by an attacker. To be 100% sure you should also compare the data of an event received from the service in a webhook notifcation with the data of an event received in a HTTP request above. The data should be the same.
You can use the code below for testing purposes:
<?php
// parse webhook notification
$body = file_get_contents('php://input');
$json = json_decode($body);
// extract application mode
$application_mode = $json->{'app_mode'};
// extract event id
$event_id = $json->{'event_id'};
// choose proper private token
switch ($application_mode) {
case "TEST":
$private_token = 'PASTE_YOUR_PRIVATE_TEST_TOKEN_HERE';
break;
case "LIVE":
$private_token = 'PASTE_YOUR_PRIVATE_LIVE_TOKEN_HERE';
break;
}
// send a HTTP request to the service's server
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => "{{< apiSite >}}/api/v2/webhook_events/$event_id",
CURLOPT_RETURNTRANSFER => 1,
CURLOPT_HTTPHEADER => array("Private-Token: $private_token")));
curl_exec($curl);
$status = curl_getinfo($curl)['http_code'];
curl_close($curl);
// does event with given event_id exist on Taxamo server?
if ($status == 200) {
echo "Webhook event with id $event_id was found on Taxamo server";
// You may perform additional checks here
} else {
echo "Webhook event with id $event_id was not found on Taxamo server!";
}
?>
Testing webhooks
We will try two approaches. In the first case we will use a publicly available echo service. The second assumes that you already run a server with PHP support.
1. Use echo HTTP server
Make sure that your account is in TEST
mode. Create a transaction in the service, input the sample data and confirm it.
From the webhook configuration page click the Browse webhook delivery logs link.
The first entry (at the top of the screen) should show details of a HTTP request sent from Taxamo to the Httpbin server.
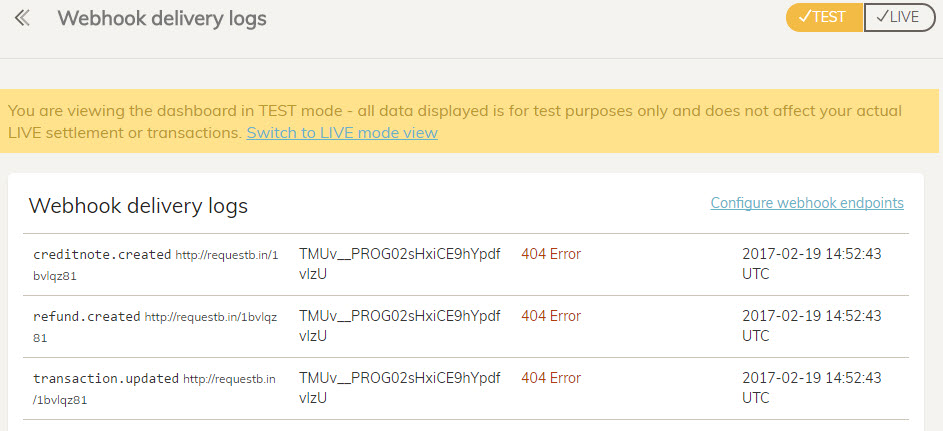
Click the line to reveal the details of the webhook delivery:
404 error
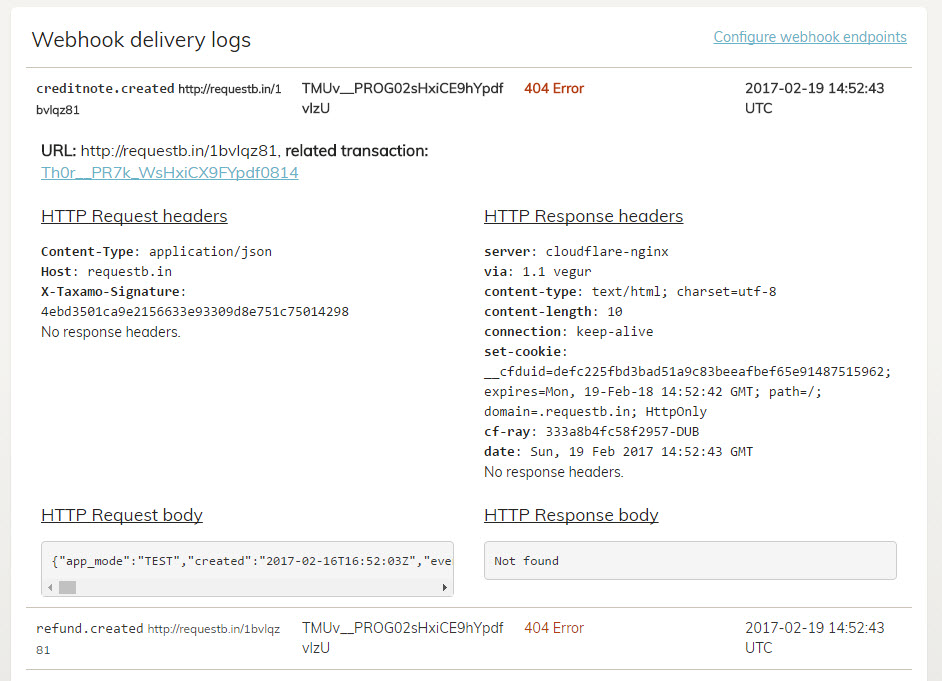
200 OK
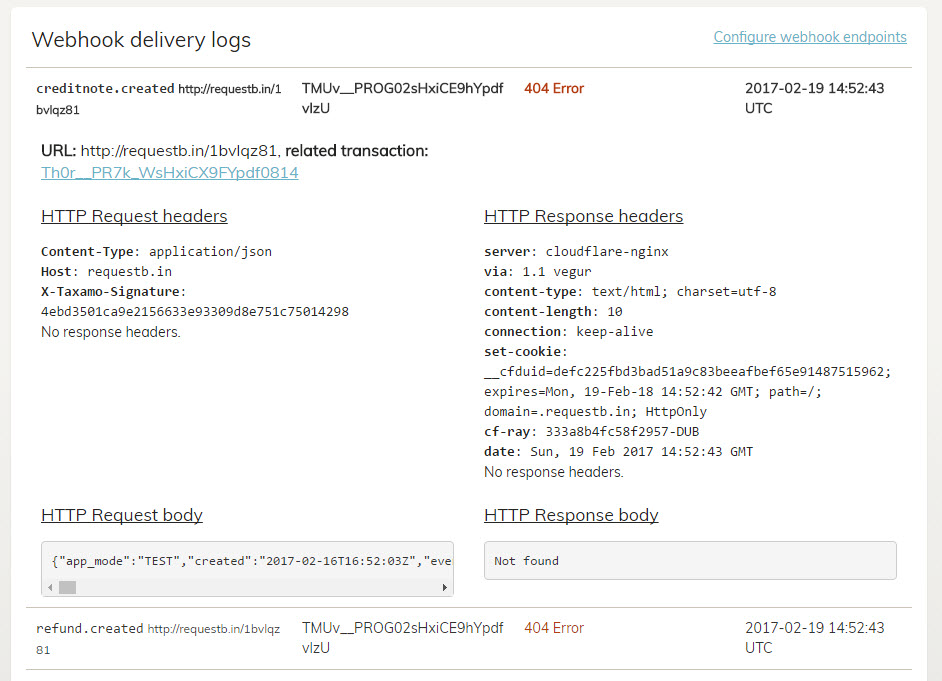
2. Use your own PHP server
Update the URL of the webhook in the Settings > Webhooks screen. It should point to a HTTP endpoint on your server:
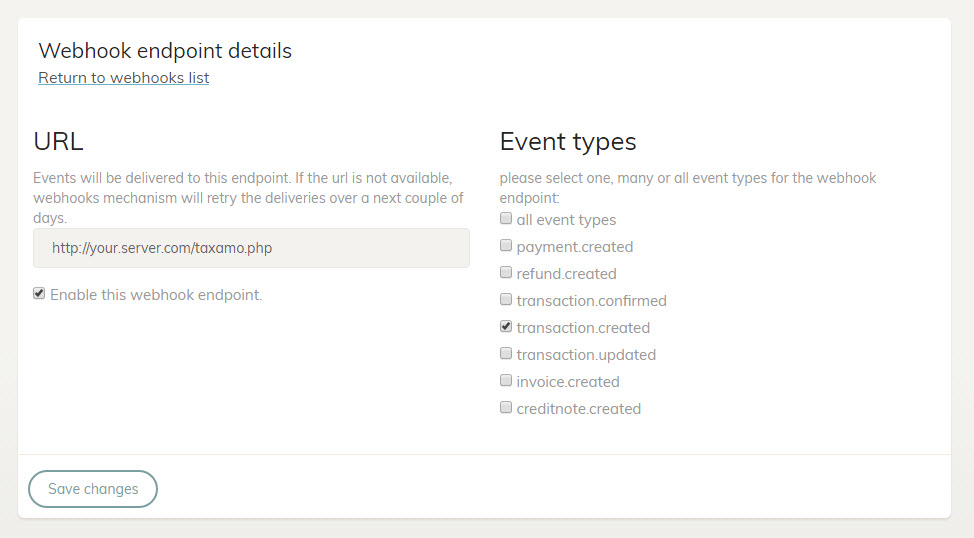
For testing purposes you can use the code snippet below. It parses the request from the Taxamo server and sends a response.
<?php
// parse request
$json = json_decode(json_decode(file_get_contents('php://input')));
// webhook processing logic here
// generate response
header('Content-Type: application/json');
$amount = $json->{'transaction'}->{'amount'};
$created = $json->{'transaction'}->{'create_timestamp'};
$arr = array('created' = $created, 'amount' = $amount);
print json_encode($arr, JSON_PRETTY_PRINT);
?>
Complete test payment with the Taxamo Checkout Form.
Wait a few seconds, then check the Settings > Webhooks > Browse webhook delivery logs page:
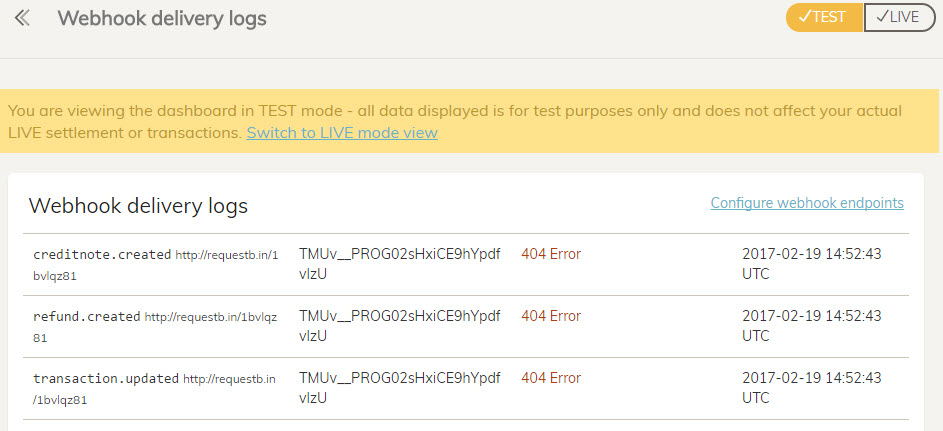
Updated almost 2 years ago